API¶
The base URL for the API is https://mht.uzi.uni-halle.de/client-seitige-web-anwendungen/api/YOUR_ID
. You must
replace the YOUR_ID
part of the URL with your student number (or any other unique ID).
The API implements validity checking of the submitted data, but it does not implement any authorisation checking. Your application must thus ensure in its code that users who do not have the necessary rights cannot modify the data.
Endpoints¶
For each object type, the endpoint documents the structure of a single instance of the object in JSONAPI format. This is the format in which POST and PATCH requests expect data to be sent to the API and the format in which GET, POST, and PATCH requests return data.
For PATCH requests, only those fields that have changed need to be included. Any fields that are not specified are left as they are by the API.
The URLs specified in this section are added to the base URL to create the final API endpoints.
Data Model¶
The basic data-model follows the following structure:
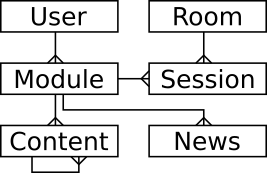
For each of the six classes there is one endpoint. Details for the attributes and relationships for each class are documented in the specific endpoints
Administrative¶
POST /reset-db
¶
Clears the developer-specific (identified via YOUR_ID
in the URL) instance of the database.
Content¶
The Content
represents textual content that is attached to the Module
. Content
can be structured hierarchically
via the parent
relationship. A Content
with no parent
specified is a top-level content that is contained
directly by the Module
. Content
objects are always according to the order
attribute.
Data Model¶
{
"type": "contents",
"id": "STRING - Unique identifier for the content",
"attributes": {
"title": "STRING - Must be at least four characters long",
"content": "STRING",
"order": "INT"
},
"relationships": {
"module": {
"data": {
"type": "modules",
"id": "STRING - The unique identifier of the module the content belongs to"
}
},
"parent": {
"data": {
"type": "contents",
"id": "STRING - The unique identifier of the parent content for hierarchical structures"
}
},
"children": {
"data": [
{
"type": "contents",
"id": "STRING - The unique identifier of the child content for hierarchical structures"
}
"The above structure can appear 0..n times"
]
}
}
}
The parent
and child
relationships are optional.
GET /contents
¶
Retrieves a list of all content.
POST /contents
¶
Creates a new content based on the content of the request’s body. For new content, the id
attribute does not have to be
set. If the content object sent with the request is valid, returns the newly created content with the id
set to its newly
created value.
GET /contents/:id
¶
Retrieves the content with the id value specified by the :id
dynamic URL segment.
PATCH /contents/:id
¶
Updates the content identified by the id value specified by the :id
dynamic URL segment to the content of the request’s
body, if the body is a valid object.
DELETE /contents/:id
¶
Deletes the content with the id value specified by the :id
dynamic URL segment.
Module¶
The Module
represents the primary object within the API. Each Module
contains zero or more Session
,
News
, and Content
. These can be empty. The only relationship that must be set is the creator
relationship,
which points to the “Teacher” User
who created the Module
.
Data Model¶
{
"type": "modules",
"id": "STRING - Unique identifier for the module",
"attributes": {
"code": "STRING - Must be at least four characters long",
"name": "STRING - Must be at least four characters long",
"semester": "STRING - Must be one of SS18, WS18/19, SS19, WS19/20"
},
"relationships": {
"teacher": {
"data": {
"type": "users",
"id": "STRING - Must be the unique identifier of a valid user"
}
},
"students": {
"data": [
{
"type": "users",
"id": "STRING - Must be the unique identifier of a valid user"
},
"The above object can occurr 0..n times"
]
}
},
"news": {
"data": [
{
"type": "news",
"id": "STRING - Must be the unique identifier of a valid news"
},
"The above object can occur 0..n times."
]
},
"sessions": {
"data": [
{
"type": "sessions",
"id": "STRING - Must be the unique identifier of a valid session"
},
"The above object can occur 0..n times."
]
},
"contents": {
"data": [
{
"type": "contents",
"id": "STRING - Must be the unique identifier of a valid content"
},
"The above object can occurr 0..n times."
]
}
}
}
The news
, sessions
, and contents
relationships are optional.
GET /modules
¶
Retrieves a list of all modules.
POST /modules
¶
Creates a new module based on the content of the request’s body. For new modules, the id
attribute does not have to
be set. If the room object sent with the request is valid, returns the newly created module with the id
set to its
newly created value.
GET /modules/:id
¶
Retrieves the module with the id value specified by the :id
dynamic URL segment.
PATCH /modules/:id
¶
Updates the module identified by the id value specified by the :id
dynamic URL segment to the content of the
request’s body, if the body is valid.
DELETE /modules/:id
¶
Deletes the module with the id value specified by the :id
dynamic URL segment.
News¶
The News
represents a news item that is linked to a Module
. News
objects are always ordered by their
date
attribute.
Data Model¶
{
"type": "news",
"id": "STRING - Unique identifier for the news",
"attributes": {
"title": "STRING - Must be at least four characters long",
"date": "DATE - Must be a valid ISO8601 date-time",
"text": "STRING"
},
"relationships": {
"module": {
"data": {
"type": "modules",
"id": "STRING - Must be the unique identifier of a valid module"
}
}
}
}
GET /news
¶
Retrieves a list of all news.
POST /news
¶
Creates a new news item based on the content of the request’s body. For new news items, the id
attribute does not
have to be set. If the news item object sent with the request is valid, returns the newly created news item with the
id
set to its newly created value.
GET /news/:id
¶
Retrieves the news item with the id value specified by the :id
dynamic URL segment.
PATCH /news/:id
¶
Updates the news item identified by the id value specified by the :id
dynamic URL segment to the content of the
request’s body, if the body is a valid object.
DELETE /news/:id
¶
Deletes the news item with the id value specified by the :id
dynamic URL segment.
Room¶
The Room
represents a single room that can be used in a Session
. The Room
, unlike the Module
, is
independent of the User
Data Model¶
{
"type": "rooms",
"id": "STRING - Unique identifier for the room",
"attributes": {
"name": "STRING - Must be at least four characters long",
"address": "STRING - Must be at least four characters long",
"capacity": "STRING",
"features": "STRING"
},
"relationships": {
"sessions": {
"data": [
{
"type": "sessions",
"id": "STRING - The unique identifier of a session"
},
"The above structure can occur 0..n times."
]
}
}
}
The sessions
relationship is read-only. Modifying it will have no effect on the Session
objects. However,
deleting the Room
will also delete all Session
that are linked to the Room
.
GET /rooms
¶
Retrieves a list of all rooms.
POST /rooms
¶
Creates a new room based on the content of the request’s body. For new rooms, the id
attribute does not have to be
set. If the room object sent with the request is valid, returns the newly created room with the id
set to its newly
created value.
GET /rooms/:id
¶
Retrieves the room with the id value specified by the :id
dynamic URL segment.
PATCH /rooms/:id
¶
Updates the room identified by the id value specified by the :id
dynamic URL segment to the content of the request’s
body, if the body is a valid object.
DELETE /rooms/:id
¶
Deletes the room with the id value specified by the :id
dynamic URL segment.
Session¶
The Session
represents a single teaching session for the Module
. It is linked to both the Module
and the
Room
in which it takes place. Session
are always ordered according to the date
property.
Data Model¶
{
"type": "sessions",
"id": "STRING - Unique identifier for the session",
"attributes": {
"title": "STRING - Must be at least four characters long",
"date": "DATE - Must be a valid ISO8601 date-time",
"keywords": "STRING"
},
"relationships": {
"module": {
"data": {
"type": "modules",
"id": "STRING - Must be the unique identifier of a valid module"
}
},
"room": {
"data": {
"type": "rooms",
"id": "STRING - Must be the unique identifier of a valid room"
}
}
}
}
GET /sessions
¶
Retrieves a list of all sessions.
POST /sessions
¶
Creates a new session based on the content of the request’s body. For new session, the id
attribute does not have
to be set. If the session object sent with the request is valid, returns the newly created session with the id
set
to its newly created value.
GET /sessions/:id
¶
Retrieves the session with the id value specified by the :id
dynamic URL segment.
PATCH /sessions/:id
¶
Updates the session identified by the id value specified by the :id
dynamic URL segment to the content of the
request’s body, if the body is a valid object.
DELETE /sessions/:id
¶
Deletes the session with the id value specified by the :id
dynamic URL segment.
User¶
Data Model¶
{
"type": "users",
"id": "STRING - Unique identifier for the user",
"attributes": {
"email": "STRING - Must be unique. Must be a valid e-mail address.",
"password": "STRING [optional] - The password is never returned by the API.",
"first-name": "STRING - Must be at least one character long.",
"last-name": "STRING - Must be at least one character long.",
"role": "STRING - Must be either 'teacher' or 'student'."
},
"relationships": {
"teaches": {
"data": [
{
"type": "modules",
"id": "STRING - Must be the unique identifier of a valid module. This should only be set or
read if the user is of the type 'teacher'"
},
"The above structure can occur 0..n times."
]
},
"takes": {
"data": [
{
"type": "modules",
"id": "STRING - Must be the unique identifier of a valid module. This should only be set or
read if the user is of the type 'student'"
},
"The above structure can occur 0..n times."
]
}
}
}
GET /users
¶
Retrieves a list of all users. Filtering by email and password can be used to implement checking if a user can log in with that combination.
POST /users
¶
Creates a new user based on the content of the request’s body. For new users, the id
attribute does not have to be
set. If the user object sent with the request is valid, returns the newly created room with the id
set to its newly
created value. The password
attribute must be set.
GET /users/:id
¶
Retrieves the user with the id value specified by the :id
dynamic URL segment.
PATCH /users/:id
¶
Updates the user identified by the id value specified by the :id
dynamic URL segment to the content of the request’s
body, if the body is a valid object. If the password
attribute is set, then the user’s password is updated,
otherwise it remains unchanged.
DELETE /users/:id
¶
Deletes the user with the id value specified by the :id
dynamic URL segment.
Filtering¶
Any GET
request to a collection URL (/modules
, /rooms
, …) can be filtered by passing the filters as
property=value
pairs as the query part of the request.
For example GET /modules?semester=WS18
returns all modules where the semester
property is set to “WS18”.